# Big numbers support
WARNING
Big numbers support has been added in the vREST NG application from v2.2.7. This feature support is in the beta stage.
# Problem:
JSON the format has no trouble in representing high-precision numbers (and big integers). But Javascript implementations of JSON (including the one now built into the language) typically represent JSON numbers as Javascript numbers locally (in theory, they could implement their own high-precision numbers/bignums). So in these applications, whatever information may have been in the JSON format is reduced to what can be represented in Javascript's IEEE 754 double-precision number format (i.e., 53 bits of precision).
For more information on the problem statement, please read this post.
So, in Javascript, if you parse the JSON string having high-precision numbers and big numbers then those numbers will be rounded off.
# Solution:
In the vREST NG Application, you may enable the big numbers to support by visiting the Configuration tab
>> Miscellaneous Section
. And then you may enable the big numbers support as shown in the below image. This is a project-level setting, So this flag will enable the support only for the current project.
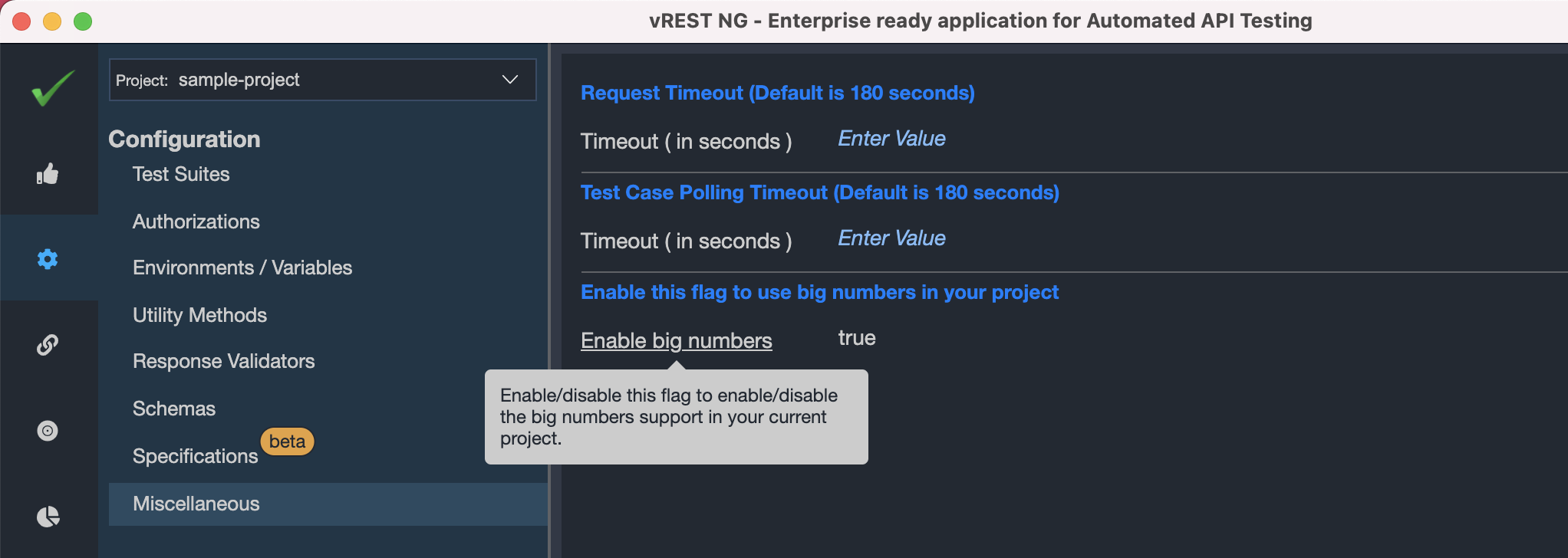
Once you enable this flag, then you will be able to define high-precision numbers and big numbers without any issue throughout the application.
vREST big number format: vREST NG will internally convert the big numbers to a string prefixed with BigInt::
.
e.g. the number 3812690672836748001
will be converted to "BigInt::3812690672836748001"
internally. We will call this representation as vREST big number format
throughout this guide.
While sending the API request, it will again convert all such big numbers formatted in vREST big number format to number before sending. Special care must be given when using the big numbers in some situations to prevent the loss of information. So, let's see how you may use the big numbers throughout the vREST NG Application.
In
Global Variables
section:In the
Global Variables
section, you may define the big numbers by simply providing the big number value and setting its type to number.Similarly you may define a JSON object containing big numbers and set it's type to object. For more information, refer the screenshot below:
In
Utility Methods
section:In
utility methods
, if you are passing some big numbers as parameters then you will receive such numbers in vREST big number format. And if you want to return a big number from the utility method then you will need to return it in vREST big number format, otherwise, the value may be truncated/rounded off.vREST provides some special methods to handle the big numbers in utility methods. We recommend you use those methods in your utility methods so that if our implementation changes in the future then you won't need to change anything in your API tests.
Inside utility methods, you may invoke these special methods using the syntax below:
this.methods.METHOD_NAME(METHOD_ARGUMENTS...)
e.g.
this.methods.getBigNumber("3812690672836748001")
And in your API tests, you may invoke these special methods using the syntax below:
{{METHOD_NAME(METHOD_ARGUMENTS...)}}
e.g.
{{getBigNumber("3812690672836748001")}}
vREST provides the following special methods.
getBigNumber(value: string | number | bigint, returnDecimal?: boolean): any
:This method will convert the big number to vREST big number format if the
returnDecimal
parameter is not set or set as false.If you want to perform some arithmetic operations on the big numbers inside the utility methods then set the second parameter
returnDecimal
to true. Then, in this case, vREST will return an object of decimal.js library. You are free to use any other big number library as per your needs if you don't want to use thedecimal.js
.isBigNumber(value: any): boolean
:This method will check whether the given input value is in vREST big number format or not. If the value is in vREST big number format then this method will return true otherwise it will return false.
getBigNumberString(value: any): string
:This method will convert the input value given in vREST big number format to a normal string. e.g. input value
"BigInt::3812690672836748001"
will converted to"3812690672836748001"
.parseJSON(str: string, reviver): any
:This method is a substitute for the
JSON.parse()
method given byJavascript
with big number support. If you parse an object string having big numbers using theJSON.parse
method then big numbers will be rounded off in the returned object without any exception. But the above methodparseJSON
will format any such big numbers in the object string to vREST big number format. e.g.If you have the following JSON string:
{ "number": 3812690672836748001 }
And if you parse the above string through
JSON.parse
then you will get the following object:{ "number": 3812690672836748000 }
But if you parse the above JSON string through vREST provided
parseJSON
method then you will get the following object as per the current implementation:{ "number": "BigInt::3812690672836748001" }
stringifyJSON(obj, replacer?, space?): string
:This method is a substitute for the
JSON.stringify()
method given byJavascript
with big number support. It will help you to stringify a JSON object having big numbers in vREST big number format to a proper JSON string. e.g.If you have the following JSON object:
{ "number": "BigInt::3812690672836748001" }
And if you stringify the above JSON object through vREST provided
stringifyJSON
method then you will get the following string as per the current implementation:{ "number": 3812690672836748001 }
In
API Tests
:In API tests, if you are using a global variable or if you are invoking a utility method that is returning a big number in vREST big number format then vREST will automatically format it to a number before sending the actual request. And if you want to use the hard-coded big number in your API tests, then you may provide the value as a normal number. vREST will automatically take care of the rest.